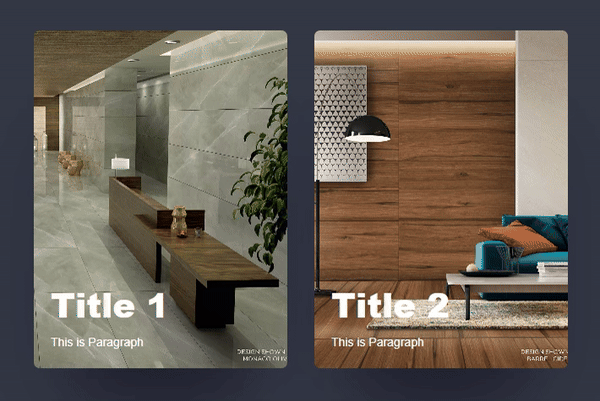
Image Card Animation
Here, we create a image card animation using HTML, CSS and JS.
Project Folder Structure
Before we start coding we take a look at the project folder structure. We start by creating a folder called – “image card animation” Inside this folder, we have 3 files. Create these files like below:
- index.html
- style.css
- script.js
HTML
We create image card animation using HTML code. Copy the code below and paste it into your index.html file.
Title 1
This is Paragraph
Title 2
This is Paragraph
CSS
We create image card animation using CSS code. Copy the code below and paste it into your style.css file.
:root {
--cover-timing: 0.5s;
--cover-ease: cubic-bezier(0.66, 0.08, 0.19, 0.97);
--cover-stagger: 0.15s;
--text-timing: 0.75s;
--text-stagger: 0.015s;
--text-ease: cubic-bezier(0.38, 0.26, 0.05, 1.07);
--title-stagger: 0.05s;
--highlight: white;
}
.card {
position: relative;
overflow: hidden;
aspect-ratio: 0.75;
display: flex;
flex-direction: column;
border-radius: 7px;
box-shadow: rgba(255, 255, 255, 0.3) 0 5vw 6vw -8vw, rgba(255, 255, 255, 0) 0 4.5vw 5vw -6vw, rgba(50, 50, 80, 0.5) 0px 4vw 8vw -2vw, rgba(0, 0, 0, 0.8) 0px 4vw 5vw -3vw;
transition: box-shadow 1s var(--cover-ease);
}
.card > * {
z-index: 2;
}
.card > img {
z-index: 0;
transition: all 0.8s cubic-bezier(0.66, 0.08, 0.19, 0.97);
}
.card::before, .card::after {
content: "";
width: 100%;
height: 50%;
top: 0;
left: 0;
background: rgba(0, 0, 0, 0.5);
position: absolute;
transform-origin: left;
transform: scaleX(0);
transition: all var(--cover-timing) var(--cover-ease);
z-index: 1;
}
.card::after {
transition-delay: var(--cover-stagger);
top: 50%;
}
.card:hover, .card:focus {
box-shadow: white 0 5vw 6vw -9vw, var(--highlight) 0 5.5vw 5vw -7.5vw, rgba(50, 50, 80, 0.5) 0px 4vw 8vw -2vw, rgba(0, 0, 0, 0.8) 0px 4vw 5vw -3vw;
}
.card:hover::before, .card:focus::before, .card:hover::after, .card:focus::after {
transform: scaleX(1);
}
.card:hover h2 .char, .card:focus h2 .char, .card:hover p .word, .card:focus p .word {
opacity: 1;
transform: translateY(0);
color: inherit;
}
.card:hover h2 .char, .card:focus h2 .char {
transition-delay: calc(0.1s + var(--char-index) * var(--title-stagger));
}
.card:hover p .word, .card:focus p .word {
transition-delay: calc(0.1s + var(--word-index) * var(--text-stagger));
}
.card:hover img, .card:focus img {
transform: scale(1.1);
}
.card:nth-of-type(1) {
--highlight: coral;
}
.card:nth-of-type(2) {
--highlight: #56ffe5;
}
.text {
position: absolute;
inset: 20px;
top: auto;
}
h2 {
font-size: 30px;
font-size: clamp(20px, 4vw, 40px);
font-weight: 800;
margin-bottom: 0.2em;
}
p {
font-size: 12px;
font-size: clamp(10px, 1.25vw, 14px);
line-height: 1.4;
text-align: justify;
margin-top: 0.2em;
margin-bottom: 0;
}
h2 .char, p .word {
color: var(--highlight);
display: inline-block;
opacity: 0;
position: relative;
transform: translateY(20px);
transition-property: transform, opacity, color;
transition-timing-function: var(--text-ease);
transition-duration: var(--text-timing), var(--text-timing), calc(var(--text-timing)*2);
}
img {
position: absolute;
inset: 0;
width: 100%;
height: 100%;
object-fit: cover;
border-radius: 7px;
}
main {
grid-template-columns: 1fr;
grid-template-rows: 60px;
grid-gap: 2em;
}
@media screen and (min-width: 600px) {
main {
grid-template-columns: 1fr 1fr;
grid-template-rows: min-content 1fr;
}
}
.card {
width: 90vw;
max-width: 300px;
}
@media screen and (min-width: 600px) {
.card {
width: 40vw;
}
}
h1 {
color: #5b6377;
font-weight: 100;
}
@media screen and (min-width: 600px) {
h1 {
grid-column: 0.3333333333;
}
}
body, main {
display: grid;
place-items: center;
}
body, html {
color: white;
background: #333844;
padding: 0;
margin: 0;
min-height: 100vh;
font-family: "Open Sans", sans-serif;
}
body {
padding: 1em 0 3em;
min-height: calc(100vh - 4em);
}
JS
Finally, we add functionality in image card animation using JavaScript. Copy the code below and paste it into your script.js file.
Splitting();
setTimeout(() => {
document.querySelector(".card").focus();
},1500);
CODEPEN
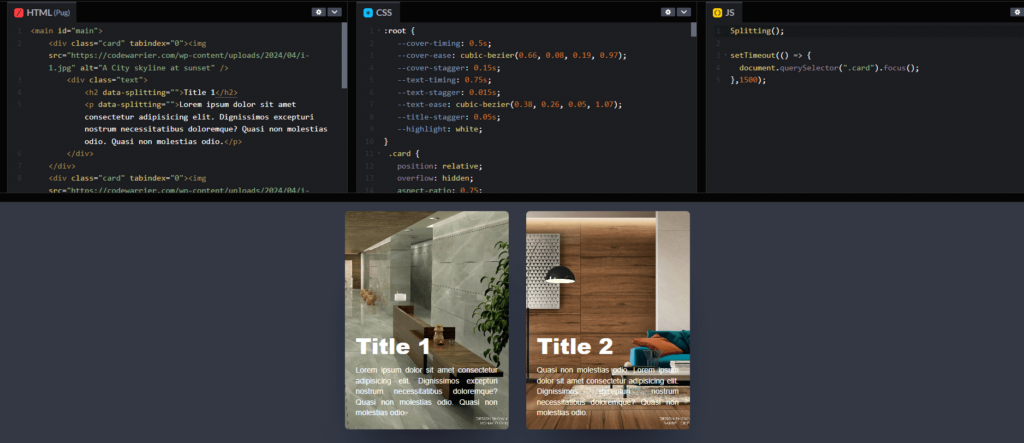
Watch Some Other Topics