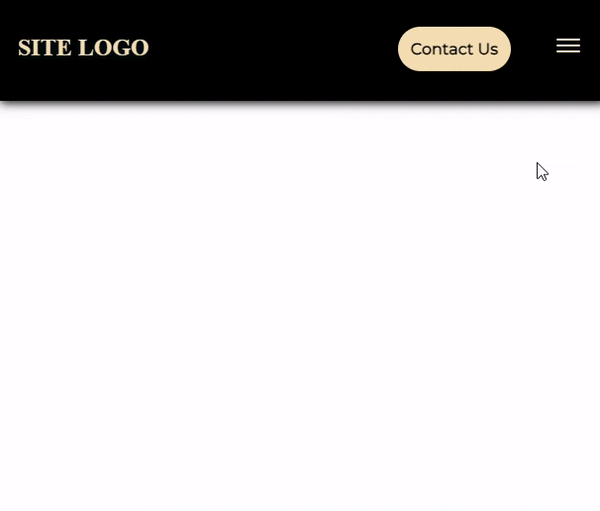
Dark Header
Here, we create a Dark Header using HTML, CSS and JS.
Project Folder Structure
Before we start coding we take a look at the project folder structure. We start by creating a folder called – “Dark Header” Inside this folder, we have 3 files. Create these files like below:
- index.html
- style.css
- script.js
HTML
We create dark header using HTML code. Copy the code below and paste it into your index.html file.
SITE LOGO
CSS
We create dark header using CSS code. Copy the code below and paste it into your style.css file.
.logo {
position: absolute;
left: 20px;
width: 200px;
cursor: pointer;
}
li, a, button{
font-family: "Montserrat", sans-serif;
font-weight: 600;
font-size: 16px;
color: bisque;
text-decoration: none;
}
header{
background-color: black;
display: flex;
justify-content: flex-end;
align-items: center;
box-shadow: 2px 2px 10px black;
}
.navlinks li{
display: inline-block;
margin: 30px;
align-items: center;
}
.navlinks li a:hover{
color: #fff;
text-shadow: 1px 1px 5px #000;
}
button{
width: 100%;
padding: 12px;
background-color: #f5deb3;
color:#000;
border-radius: 50px;
outline: none;
cursor: pointer;
transition: all 0.5s, ease 0s;
border: none;
z-index: 999;
border:1px solid transparent;
}
button:hover{
border:1px solid #fff;
background-color:transparent;
color:#fff;
}
.sidebar{
position: fixed;
top: 0;
right: 0;
height: 100vh;
width: 100%;
z-index: 999;
background-color: transparent;
backdrop-filter: blur(10px);
background-color:#000;
display: none;
justify-content: flex-start;
list-style: none;
flex-direction: column;
transition: transform 0.3s ease;
transform: translateX(100%);
}
.sidebar li{
width: 100%;
margin: 25px;
}
.sidebar img{
position: absolute;
bottom: 20px;
width: 100%;
height: 120px;
}
nav ion-icon{
font-size: 2em;
position: absolute;
top: 35px;
right: 20px;
}
h2{
color: #f5deb3;
text-shadow: #002423 -2px 2px 10px;
text-align: left;
}
@media only screen and (min-width: 830px){
.navlinks ion-icon{
display: none;
}
}
@media only screen and (max-width: 830px){
.navlinks .items{
display: none;
}
}
JS
Finally, we add functionality in dark header using JavaScript. Copy the code below and paste it into your script.js file.
function showSidebar() {
const sidebar = document.querySelector(".sidebar");
sidebar.style.display = 'flex';
setTimeout(() => {
sidebar.style.transform = 'translateX(0)';
}, 10);
}
function closeSidebar() {
const sidebar = document.querySelector(".sidebar");
sidebar.style.transform = 'translateX(100%)';
setTimeout(() => {
sidebar.style.display = 'none';
}, 300);
}
CODEPEN

Watch Some Other Topics